iOS Parser - Version 2 + Migration Guide
The iOS parsing SDK has launched a new version that contains enhanced validation.
Existing users of the IDScanPDFParser and IDScanMRZParser dependencies will need to do small development work to migrate to version 2.
The update will be needed if the major version is listed as 1.
Note
Version 2 of the iOS parser will still work with the camera scanning SDK.
Dependency Changes
In version 1, the iOS parser contained a separate library for PDF417 (IDScanPDFParser) and MRZ (IDScanMRZParser).
When updating to version 2, these dependencies are now combined into one (IDScanIDParser).
License Key Changes
License Keys on version one of the ID Parsing SDK will work on version two if the bundle ID has not changed for the project.
A feature of version 2 is enhanced validation. This is displayed into two fields of the parsed results.
validationConfidence - Confidence score of the validity of the data string.
validationCodes - List of 5 digit codes that is linked to IDScan.net validation tests.
Note
Existing users on version one interested in these fields should reach out to support@idscan.net for a key replacement with the email containing their existing PARSING key(s).
Code Changes
Methods
Method Name | Description | Return Type |
---|---|---|
setLicense(_ license: String) -> Bool | Sets the license key for the parser. A parameter of the license given from IDScan.net is passed to this method. | Bool: true if the license is set successfully, false otherwise. |
parse(_ trackString: String) -> [String: String]? | Parses the given track string and returns the parsed data as a dictionary. The trackstring of the document is passed to this method (ex from Camera Scanning SDK). | [String: Any]?: A dictionary containing the parsed data, or nil if parsing fails. |
Installation
Installing from Xcode (relevant for both Swift and Objective-C projects)
Add the package by selecting Your project name
→ Package Dependencies
→ +
.
Search for the IDScanIDParser using the repo's URL:
https://github.com/IDScanNet/IDScanIDParserIOS
Next, set the Dependency Rule
to be Up to Next Major Version
and specify the latest version of the package as the lower bound.
Then, select Add Package
.
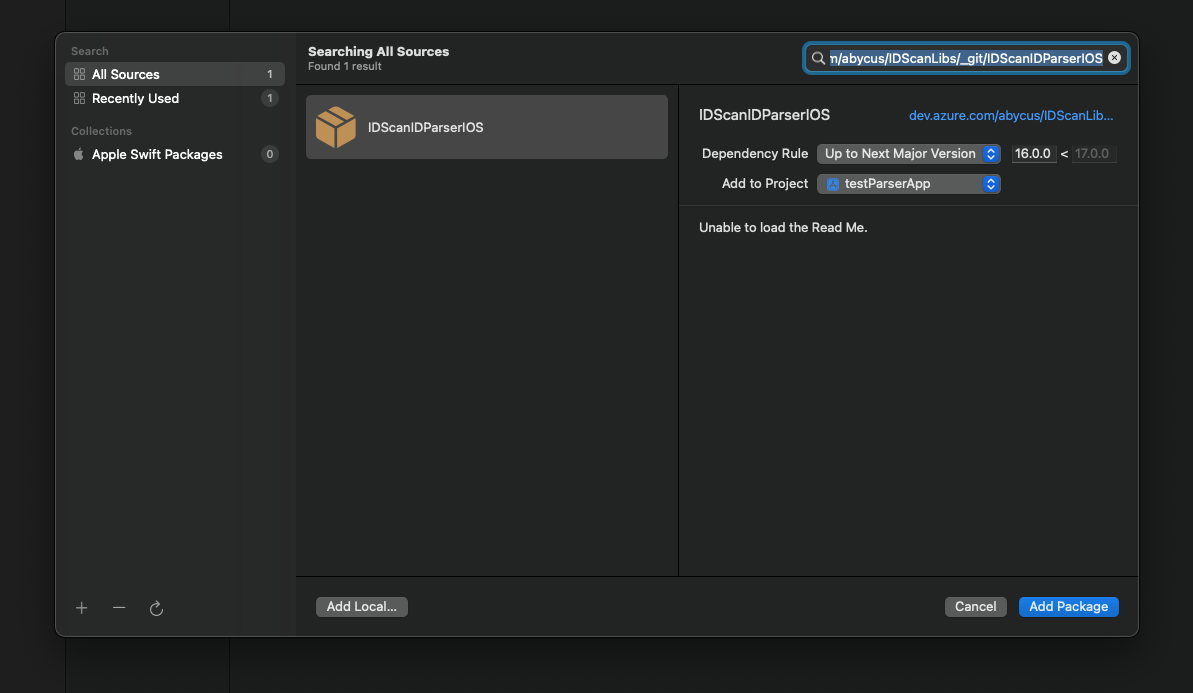
Choose the parser.
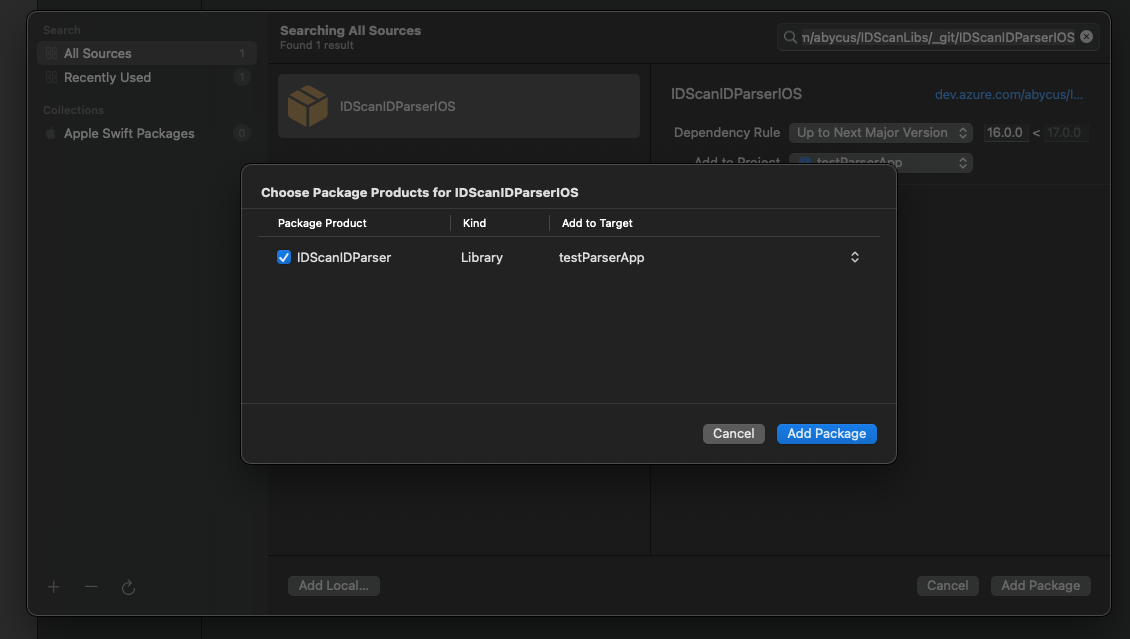
Adding IDScanIDParser to a Package.swift
For integration via a Package.swift
manifest instead of Xcode, you can add IDScanIDParser to the dependencies array of your package:
let package = Package(
name: "MyPackage",
dependencies: [
.package(url: "https://github.com/IDScanNet/IDScanIDParserIOS", .upToNextMajor(from: "2.0.0"))
],
// ...
)
Then, in any target that depends on the parsers, add it to the dependencies
array of that target:
.target(
name: "MyTarget",
dependencies: [
.product(name: "IDScanIDParser", package: "IDScanIDParserIOS")
]
),
Usage
Swift
import IDScanIDParser
// Initialize the parser
let parser = IDScanIDParser()
// Set the license key
let licenseKey = "YOUR_LICENSE_KEY"
let isLicensed = parser.setLicense(licenseKey)
if !isLicensed {
print("Wrong license.")
exit(1)
}
// Parse the track string
let trackString = "YOUR_TRACK_STRING"
if let result = parser.parse(trackString) {
print("Parsed Data: \(result)")
} else {
print("Failed to parse the track string.")
}
Objective-C
// Initialize the parser
IDScanIDParser *parser = IDScanIDParser.new;
// Set the license key
NSString *licenseKey = @"YOUR_LICENSE_KEY";
BOOL isLicensed = [parser setLicense:licenseKey];
if (!isLicensed) {
NSLog(@"Wrong license.");
exit(1);
}
// Parse the track string
NSString *trackString = @"YOUR_TRACK_STRING";
NSDictionary *result = [parser parse:trackString];
if (result) {
NSLog(@"Parsed Data: %@", result);
} else {
NSLog(@"Failed to parse the track string.");
}